Introduction
Welcome to the FTXUI documentation!
This is a brief tutorial. You are also encouraged to self-learn by reading the examples.
Short example
To build a single frame, you need create an ftxui::Element
, and display it on a ftxui::Screen
.
main.cpp
#include <iostream>
int main(void) {
hbox({
text("left") | border,
text("middle") | border | flex,
text("right") | border,
});
auto screen = Screen::Create(
Dimension::Full(),
Dimension::Fit(document)
);
Render(screen, document);
screen.Print();
return EXIT_SUCCESS;
}
std::shared_ptr< Node > Element
output
┌────┐┌────────────────────────────────────┐┌─────┐
│left││middle ││right│
└────┘└────────────────────────────────────┘└─────┘
Configure
Using CMake and find_package
Assuming FTXUI is available or installed on the system.
CMakeLists.txt
cmake_minimum_required (VERSION 3.11)
find_package(ftxui 5 REQUIRED)
project(ftxui-starter LANGUAGES CXX VERSION 1.0.0)
add_executable(ftxui-starter src/main.cpp)
target_link_libraries(ftxui-starter
PRIVATE ftxui::screen
PRIVATE ftxui::dom
PRIVATE ftxui::component # Not needed for this example.
)
Using CMake and FetchContent
If you want to fetch FTXUI using cmake:
CMakeLists.txt
cmake_minimum_required (VERSION 3.11)
include(FetchContent)
set(FETCHCONTENT_UPDATES_DISCONNECTED TRUE)
FetchContent_Declare(ftxui
GIT_REPOSITORY https://github.com/ArthurSonzogni/ftxui
GIT_TAG main # Important: Specify a version or a commit hash here.
)
FetchContent_MakeAvailable(ftxui)
project(ftxui-starter LANGUAGES CXX VERSION 1.0.0)
add_executable(ftxui-starter src/main.cpp)
target_link_libraries(ftxui-starter
PRIVATE ftxui::screen
PRIVATE ftxui::dom
PRIVATE ftxui::component # Not needed for this example.
)
Build
mkdir build && cd build
cmake ..
make
./main
List of modules.
The project is comprised of 3 modules:
- ftxui/screen defines a
ftxui::Screen
, a grid of ftxui::Pixel
.
- ftxui/dom is the main module. It defines a hierarchical set of
ftxui::Element
. An element draws something on the ftxui::Screen
. It is responsive to the size of its container.
- ftxui/component The module is required if your program needs to respond to user input. It defines a set of
ftxui::Component
. These components can be utilized to navigate using the arrow keys and/or cursor. There are several builtin widgets like checkbox/inputbox/etc to interact with. You can combine them, or even define your own custom components.
screen
This is the visual element of the program. It defines a ftxui::Screen
, which is a grid of ftxui::Pixel
. A Pixel represents a Unicode character and its associated style (bold, italic, colors, etc.). The screen can be printed as a string using ftxui::Screen::ToString()
. The following example highlights this process:
#include <iostream>
int main(void) {
auto screen = Screen::Create(Dimension::Fixed(32), Dimension::Fixed(10));
auto& pixel = screen.PixelAt(9,9);
pixel.character = U'A';
pixel.bold = true;
pixel.foreground_color = Color::Blue;
std::cout << screen.ToString();
return EXIT_SUCCESS;
}
dom
This module defines a hierarchical set of ftxui::Element
. An element manages the layout and can be responsive to the terminal dimension changes. Note the following example where this module is used to create a simple layout with a number of operators:
Example:
text("The window") | bold | color(Color::Blue),
gauge(0.5)
text("The footer")
});
document = border(document);
document = document | border.
document |= border
List of elements
The list of all elements are included and can be accessed by including the corresponding header file:
#ifndef FTXUI_DOM_ELEMENTS_HPP
#define FTXUI_DOM_ELEMENTS_HPP
#include <functional>
#include <memory>
class Node;
using Element = std::shared_ptr<Node>;
};
float right,
float down,
Element canvas(
int width,
int height, std::function<
void(Canvas&)>);
namespace Dimension {
}
}
#include "ftxui/dom/take_any_args.hpp"
#endif
Dimensions Fit(Element &, bool extend_beyond_screen=false)
Decorator bgcolor(Color)
Decorate using a background color.
Element window(Element title, Element content, BorderStyle border=ROUNDED)
Draw window with a title and a border around the element.
Element borderDouble(Element)
Draw a double border around the element.
Element focusCursorBarBlinking(Element)
Same as focus, but set the cursor shape to be a blinking bar.
Element xflex(Element)
Expand/Minimize if possible/needed on the X axis.
Element gaugeDirection(float progress, Direction direction)
Draw a high definition progress bar progressing in specified direction.
Decorator focusPositionRelative(float x, float y)
Used inside a frame, this force the view to be scrolled toward a a given position....
Element separatorStyled(BorderStyle)
Draw a vertical or horizontal separation in between two other elements.
Element xflex_grow(Element)
Expand if possible on the X axis.
std::function< Element(Element)> Decorator
Element underlinedDouble(Element)
Apply a underlinedDouble to text.
Element clear_under(Element element)
Before drawing |child|, clear the pixels below. This is useful in.
Element borderDashed(Element)
Draw a dashed border around the element.
Element separatorEmpty()
Draw a vertical or horizontal separation in between two other elements, using the EMPTY style.
Element vscroll_indicator(Element)
Display a vertical scrollbar to the right. colors.
Element separatorVSelector(float up, float down, Color unselected_color, Color selected_color)
Draw an vertical bar, with the area in between up/downcolored differently.
Element flexbox(Elements, FlexboxConfig config=FlexboxConfig())
Element nothing(Element element)
A decoration doing absolutely nothing.
Decorator size(WidthOrHeight, Constraint, int value)
Apply a constraint on the size of an element.
Element flex(Element)
Make a child element to expand proportionally to the space left in a container.
Element paragraphAlignRight(const std::string &text)
Return an element drawing the paragraph on multiple lines, aligned on the right.
std::shared_ptr< T > Make(Args &&... args)
Element xframe(Element)
Same as frame, but only on the x-axis.
Element gaugeRight(float progress)
Draw a high definition progress bar progressing from left to right.
Element focusCursorUnderlineBlinking(Element)
Same as focus, but set the cursor shape to be a blinking underline.
Element bold(Element)
Use a bold font, for elements with more emphasis.
Element separatorLight()
Draw a vertical or horizontal separation in between two other elements, using the LIGHT style.
Element spinner(int charset_index, size_t image_index)
Useful to represent the effect of time and/or events. This display an ASCII art "video".
Element borderRounded(Element)
Draw a rounded border around the element.
Decorator selectionStyle(std::function< void(Pixel &)> style)
Set the style of an element when selected.
Element yflex(Element)
Expand/Minimize if possible/needed on the Y axis.
Element flex_shrink(Element)
Minimize if needed.
Element focusCursorBar(Element)
Same as focus, but set the cursor shape to be a still block.
Element separatorHSelector(float left, float right, Color unselected_color, Color selected_color)
Draw an horizontal bar, with the area in between left/right colored differently.
Element focusCursorBlock(Element)
Same as focus, but set the cursor shape to be a still block.
Element hbox(Elements)
A container displaying elements horizontally one by one.
Element canvas(ConstRef< Canvas >)
Produce an element from a Canvas, or a reference to a Canvas.
Element underlined(Element)
Make the underlined element to be underlined.
Element center(Element)
Center an element horizontally and vertically.
Decorator selectionForegroundColor(Color foreground)
Set the foreground color of an element when selected. Note that the style is applied on top of the ex...
Element focusCursorUnderline(Element)
Same as focus, but set the cursor shape to be a still underline.
Component operator|(Component component, ComponentDecorator decorator)
Element borderHeavy(Element)
Draw a heavy border around the element.
Element inverted(Element)
Add a filter that will invert the foreground and the background colors.
Element paragraphAlignCenter(const std::string &text)
Return an element drawing the paragraph on multiple lines, aligned on the center.
Decorator selectionBackgroundColor(Color foreground)
Set the background color of an element when selected. Note that the style is applied on top of the ex...
Element gaugeUp(float progress)
Draw a high definition progress bar progressing from bottom to top.
Element text(std::wstring text)
Display a piece of unicode text.
Element align_right(Element)
Align an element on the right side.
Decorator focusPosition(int x, int y)
Used inside a frame, this force the view to be scrolled toward a a given position....
std::vector< Element > Elements
Element yframe(Element)
Same as frame, but only on the y-axis.
Element yflex_grow(Element)
Expand if possible on the Y axis.
Element hscroll_indicator(Element)
Display an horizontal scrollbar to the bottom. colors.
Element flex_grow(Element)
Expand if possible.
Element separatorDashed()
Draw a vertical or horizontal separation in between two other elements, using the DASHED style.
Element notflex(Element)
Make the element not flexible.
Element strikethrough(Element)
Apply a strikethrough to text.
Element italic(Element)
Apply a underlinedDouble to text.
Element dbox(Elements)
Stack several element on top of each other.
Decorator selectionColor(Color foreground)
Set the color of an element when selected.
Element xflex_shrink(Element)
Minimize if needed on the X axis.
Element gaugeLeft(float progress)
Draw a high definition progress bar progressing from right to left.
Element separatorCharacter(std::string)
Draw a vertical or horizontal separation in between two other elements.
Element select(Element e)
Set the child to be the one focused among its siblings.
Element vtext(std::wstring text)
Display a piece unicode text vertically.
Element borderLight(Element)
Draw a light border around the element.
Element focus(Element)
Set the child to be the one focused among its siblings.
Element paragraphAlignLeft(const std::string &text)
Return an element drawing the paragraph on multiple lines, aligned on the left.
Element selectionStyleReset(Element)
Reset the selection style of an element.
Decorator borderWith(const Pixel &)
Same as border but with a constant Pixel around the element.
Decorator reflect(Box &box)
std::function< std::vector< int >(int, int)> GraphFunction
Decorator borderStyled(BorderStyle)
Same as border but with different styles.
Element gridbox(std::vector< Elements > lines)
A container displaying a grid of elements.
Element separator()
Draw a vertical or horizontal separation in between two other elements.
Element filler()
An element that will take expand proportionally to the space left in a container.
Elements paragraph(std::wstring text)
Element dim(Element)
Use a light font, for elements with less emphasis.
Element automerge(Element child)
Enable character to be automatically merged with others nearby.
Element frame(Element)
Allow an element to be displayed inside a 'virtual' area. It size can be larger than its container....
Decorator hyperlink(std::string link)
Decorate using an hyperlink. The link will be opened when the user click on it. This is supported onl...
Element blink(Element)
The text drawn alternates in between visible and hidden.
Element vcenter(Element)
Center an element vertically.
Element separatorDouble()
Draw a vertical or horizontal separation in between two other elements, using the DOUBLE style.
Element focusCursorBlockBlinking(Element)
Same as focus, but set the cursor shape to be a blinking block.
Component & operator|=(Component &component, ComponentDecorator decorator)
Element gauge(float progress)
Draw a high definition progress bar.
Element paragraphAlignJustify(const std::string &text)
Return an element drawing the paragraph on multiple lines, aligned using a justified alignment....
Element graph(GraphFunction)
Draw a graph using a GraphFunction.
Element border(Element)
Draw a border around the element.
Element separatorHeavy()
Draw a vertical or horizontal separation in between two other elements, using the HEAVY style.
Element borderEmpty(Element)
Draw an empty border around the element.
Decorator color(Color)
Decorate using a foreground color.
Element yflex_shrink(Element)
Minimize if needed on the Y axis.
Element hcenter(Element)
Center an element horizontally.
Element vbox(Elements)
A container displaying elements vertically one by one.
Element gaugeDown(float progress)
Draw a high definition progress bar progressing from top to bottom.
text
The most simple widget. It displays a text.
text("I am a piece of text");
vtext
Identical to ftxui::text
, but displayed vertically.
Code:
Terminal output:
paragraph
Similar to ftxui::text
, but the individual word are wrapped along multiple lines, depending on the width of its container.
Sample Code:
paragraph("A very long text")
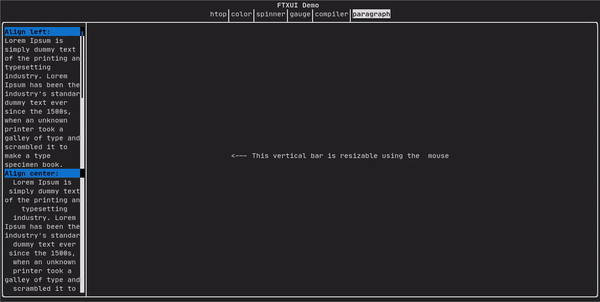
For a more detailed example refer to detailed example. Paragraph also includes a number of other variants as shown below:
Element paragraph(std::string text);
Element paragraphAlignLeft(std::string text);
Element paragraphAlignRight(std::string text);
Element paragraphAlignCenter(std::string text);
Element paragraphAlignJustify(std::string text);
border
Adds a border around an element.
Code:
border(text("The element"))
Terminal output:
┌───────────┐
│The element│
└───────────┘
You can achieve the same behavior by using the pipe operator.
Code:
text("The element") | border
Border also comes in a variety of styles as shown below:
A Unicode character and its associated style.
window
A ftxui::window
is a ftxui::border
, but with an additional header. To add a window around an element, wrap it and specify a string as the header. Code:
window("The window", text("The element"))
Terminal output:
┌The window─┐
│The element│
└───────────┘
separator
Displays a vertical/horizontal line to visually split the content of a container in two.
Code:
border(
hbox({
text("Left"),
separator(),
text("Right")
})
)
Terminal output:
┌────┬─────┐
│left│right│
└────┴─────┘
Separators come in a variety of flavors as shown below:
Element separatorCharacter(std::string);
Element separatorHSelector(
float left,
float right,
Element separatorVSelector(
float up,
float down,
A class representing terminal colors.
gauge
It constitutes a gauge. It can be used to represent a progress bar.
Code:
Teminal output:
┌────────────────────────────────────────────────────────────────────────────┐
│██████████████████████████████████████ │
└────────────────────────────────────────────────────────────────────────────┘
Gauges can be displayed in many orientations as shown below:
Element gaugeDirection(
float ratio, GaugeDirection);
graph
See:
Colors
Most terminal consoles can display colored text and colored backgrounds. FTXUI supports every color palette:
Color gallery: 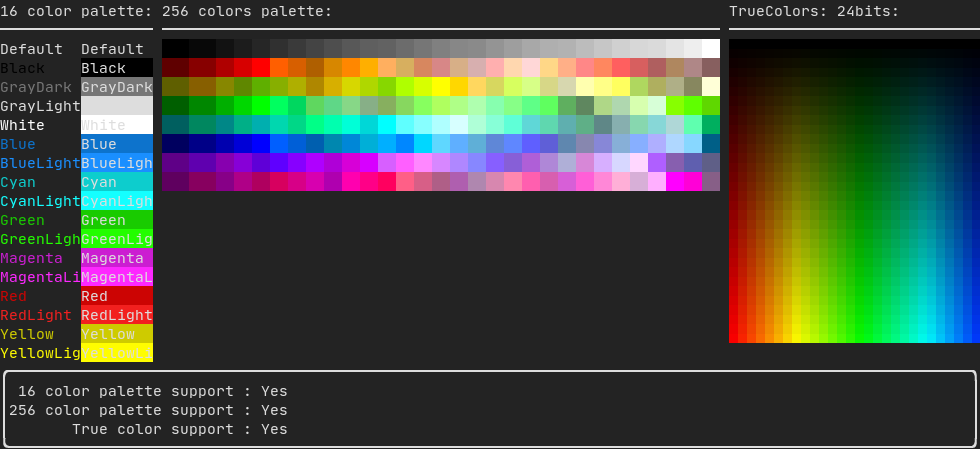
Palette16
On most terminals the following colors are supported:
- Default
- Black
- GrayDark
- GrayLight
- White
- Blue
- BlueLight
- Cyan
- CyanLight
- Green
- GreenLight
- Magenta
- MagentaLight
- Red
- RedLight
- Yellow
- YellowLight
Example use of the above colors using the pipe operator:
text("Blue foreground") | color(Color::Blue);
text("Blue background") | bgcolor(Color::Blue);
text("Black on white") | color(Color::Black) | bgcolor(Color::White);
Palette256
On terminal supporting 256 colors.
text("HotPink") | color(Color::HotPink);
TrueColor
On terminal supporting trueColor, you can directly use the 24bit RGB color space:
Use the constructors below to specify the RGB or HSV values for your color:
There are two constructors:
static Color HSV(uint8_t hue, uint8_t saturation, uint8_t value)
Build a Color from its HSV representation. https://en.wikipedia.org/wiki/HSL_and_HSV.
static Color RGB(uint8_t red, uint8_t green, uint8_t blue)
Build a Color from its RGB representation. https://en.wikipedia.org/wiki/RGB_color_model.
LinearGradient
FTXUI supports linear gradient. Either on the foreground or the background.
A class representing the settings for linear-gradient color effect.
A ftxui::LinearGradient
is defined by an angle in degree, and a list of color stops.
.AddStop(0.0, Color::Red)
.AddStop(0.5, Color::Green)
.AddStop(1.0, Color::Blue);
LinearGradient & Angle(float angle)
Set the angle of the gradient.
You can also use simplified constructors:
See demo.
Style
In addition to colored text and colored backgrounds. Many terminals support text effects such as: bold
, italic
, dim
, underlined
, inverted
, blink
.
Example

To use these effects, simply wrap your elements with your desired effect:
underlined(bold(text("This text is bold and underlined")))
Alternatively, use the pipe operator to chain it on your element:
text("This text is bold") | bold | underlined
Layout
Enables elements to be arranged in the following ways:
Example using ftxui::hbox
, ftxui::vbox
and ftxui::filler
.
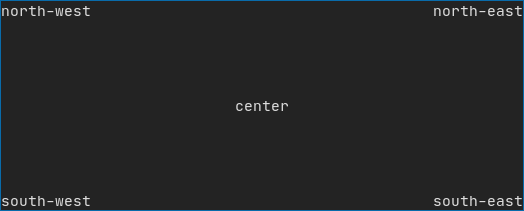
Example using ftxui::gridbox
:
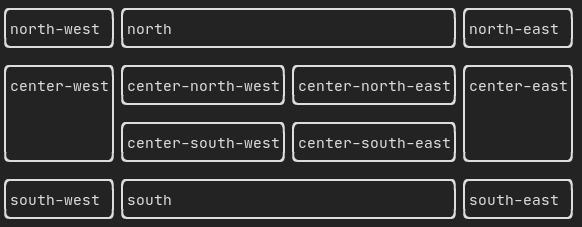
Example using flexbox:
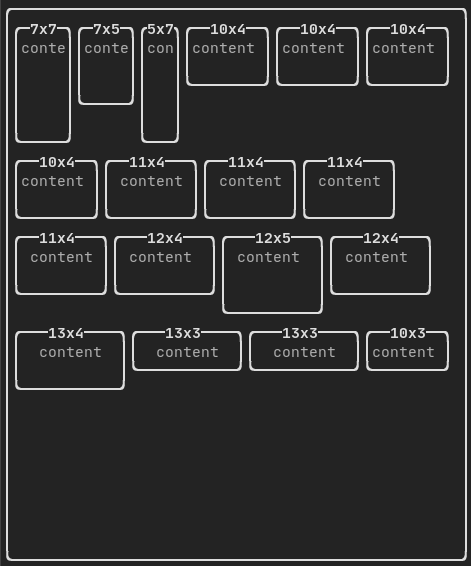
Checkout this example and the associated demo.
Element can also become flexible using the the ftxui::flex
decorator.
Code:
hbox({
text("left") | border ,
text("middle") | border | flex,
text("right") | border,
});
Terminal output:
┌────┐┌─────────────────────────────────────────────────────┐┌─────┐
│left││middle ││right│
└────┘└─────────────────────────────────────────────────────┘└─────┘
Code:
hbox({
text("left") | border ,
text("middle") | border | flex,
text("right") | border | flex,
});
Terminal output:
┌────┐┌───────────────────────────────┐┌───────────────────────────────┐
│left││middle ││right │
└────┘└───────────────────────────────┘└───────────────────────────────┘
Table
Enables easy formatting of data into a neat table like visual form.
Code example:
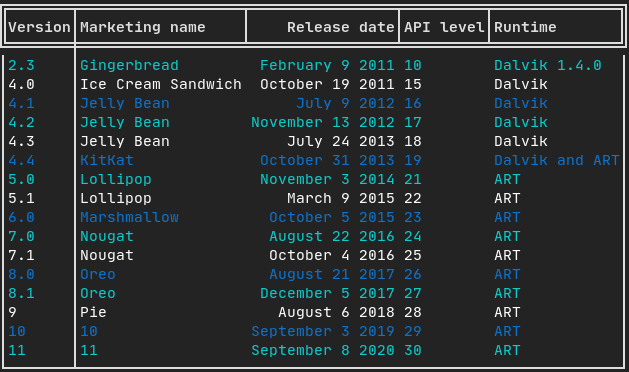
Canvas
See the API <ftxui/dom/canvas.hpp>
c.DrawPointLine(10, 10, 80, 10, Color::Red);
auto element = canvas(c);
Drawing can be performed on a ftxui::Canvas
, using braille, block, or simple characters:
Simple example:
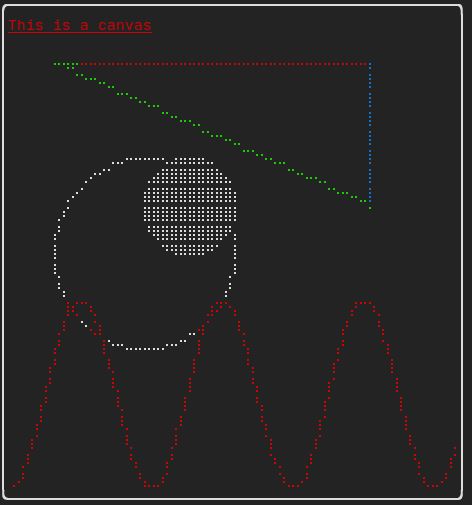
Complex example:
component
The ftxui::component
module defines the logic that produces interactive components that respond to user events (keyboard, mouse, etc.).
A ftxui::ScreenInteractive
defines a main loop that renders a component.
A ftxui::Component
is a shared pointer to a ftxui::ComponentBase
. The latter defines:
ftxui::Element
are used to render a single frame.
ftxui::Component
are used to render dynamic user interface, producing multiple frame, and updating its state on events.
Gallery of multiple components. (demo)
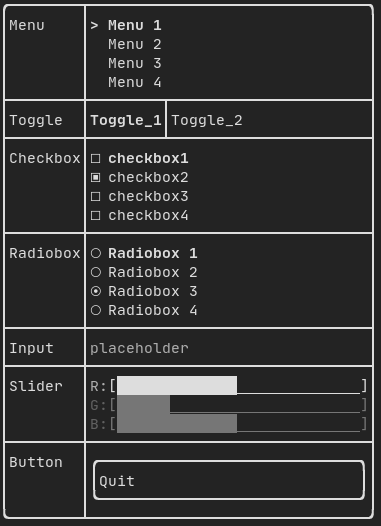
All predefined components are available in "ftxui/dom/component.hpp"
#ifndef FTXUI_COMPONENT_HPP
#define FTXUI_COMPONENT_HPP
#include <functional>
#include <memory>
#include <utility>
struct ButtonOption;
struct CheckboxOption;
struct Event;
struct InputOption;
struct MenuOption;
struct RadioboxOption;
struct MenuEntryOption;
template <
class T,
class...
Args>
return std::make_shared<T>(std::forward<Args>(
args)...);
}
namespace Container {
}
std::function<void()> on_click,
bool* checked,
StringRef placeholder,
int* selected_,
int* selected_,
template <typename T>
Ref<int> value,
std::function<void()> on_enter,
std::function<void(bool)> on_change);
}
#endif
Component Horizontal(Components children)
A list of components, drawn one by one horizontally and navigated horizontally using left/right arrow...
Component Vertical(Components children)
A list of components, drawn one by one vertically and navigated vertically using up/down arrow key or...
Component Stacked(Components children)
A list of components to be stacked on top of each other. Events are propagated to the first component...
Component Tab(Components children, int *selector)
A list of components, where only one is drawn and interacted with at a time. The |selector| gives the...
Component Maybe(Component, const bool *show)
Decorate a component |child|. It is shown only when |show| is true.
Component ResizableSplitTop(Component main, Component back, int *main_size)
An vertical split in between two components, configurable using the mouse.
Component Menu(MenuOption options)
A list of text. The focused element is selected.
Component MenuEntry(MenuEntryOption options)
A specific menu entry. They can be put into a Container::Vertical to form a menu.
std::function< Element(Element)> ElementDecorator
std::shared_ptr< ComponentBase > Component
Component Toggle(ConstStringListRef entries, int *selected)
An horizontal list of elements. The user can navigate through them.
std::vector< Component > Components
Component Radiobox(RadioboxOption options)
A list of element, where only one can be selected.
Component Button(ButtonOption options)
Draw a button. Execute a function when clicked.
Component Modal(Component main, Component modal, const bool *show_modal)
Component Renderer(Component child, std::function< Element()>)
Return a new Component, similar to |child|, but using |render| as the Component::Render() event.
Component Hoverable(Component component, bool *hover)
Wrap a component. Gives the ability to know if it is hovered by the mouse.
Component ResizableSplit(ResizableSplitOption options)
A split in between two components.
Component Window(WindowOptions option)
A draggeable / resizeable window. To use multiple of them, they must be stacked using Container::Stac...
Component Input(InputOption options={})
An input box for editing text.
Component ResizableSplitRight(Component main, Component back, int *main_size)
An horizontal split in between two components, configurable using the mouse.
Component Dropdown(ConstStringListRef entries, int *selected)
A dropdown menu.
Component Slider(SliderOption< T > options)
A slider in any direction.
Component ResizableSplitBottom(Component main, Component back, int *main_size)
An vertical split in between two components, configurable using the mouse.
Component Checkbox(CheckboxOption options)
Draw checkable element.
Component ResizableSplitLeft(Component main, Component back, int *main_size)
An horizontal split in between two components, configurable using the mouse.
std::function< Component(Component)> ComponentDecorator
Component Collapsible(ConstStringRef label, Component child, Ref< bool > show=false)
Component CatchEvent(Component child, std::function< bool(Event)>)
static CheckboxOption Simple()
Option for standard Checkbox.
Input
Example:
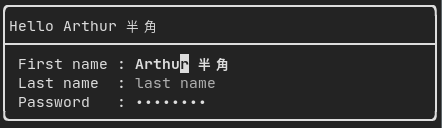
Produced by: ftxui::Input()
from "ftxui/component/component.hpp"
Filtered input
On can filter out the characters received by the input component, using ftxui::CatchEvent
.
std::string phone_number;
Component input = Input(&phone_number,
"phone number");
input |= CatchEvent([&](
Event event) {
return event.is_character() && !std::isdigit(event.
character()[0]);
});
input |= CatchEvent([&](
Event event) {
return event.is_character() && phone_number.size() >= 10;
});
Represent an event. It can be key press event, a terminal resize, or more ...
std::string character() const
Menu
Defines a menu object. It contains a list of entries, one of them is selected.
Example:

Produced by: ftxui::Menu()
from "ftxui/component/component.hpp"
Toggle
A special kind of menu. The entries are displayed horizontally.
Example:

Produced by: ftxui::Toggle()
from "ftxui/component/component.hpp"
CheckBox
This component defines a checkbox. It is a single entry that can be turned on/off.
Example:
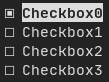
Produced by: ftxui::Checkbox()
from "ftxui/component/component.hpp"
RadioBox
A radiobutton component. This is a list of entries, where one can be turned on.
Example:
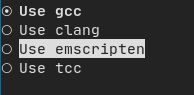
Produced by: ftxui::Radiobox()
from "ftxui/component/component.hpp"
Dropdown
A drop down menu is a component that when checked display a list of element for the user to select one.
Example:
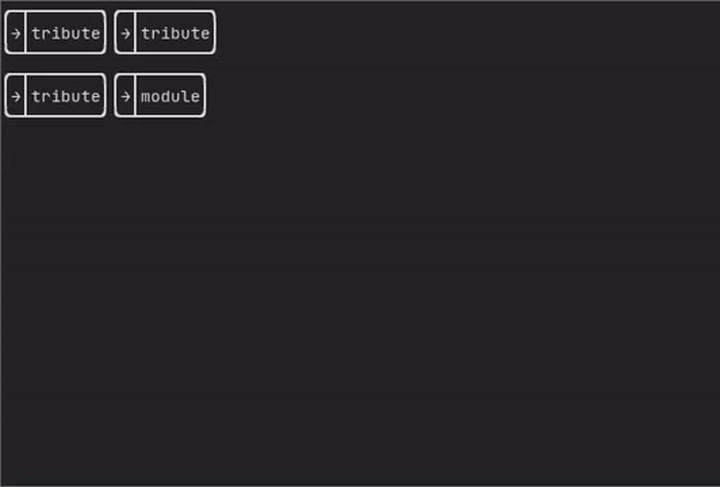
Produced by: ftxui::Dropdown()
from "ftxui/component/component.hpp"
Slider
Represents a slider object that consists of a range with binned intermediate intervals. It can be created by ftxui::Slider()
.
Example:
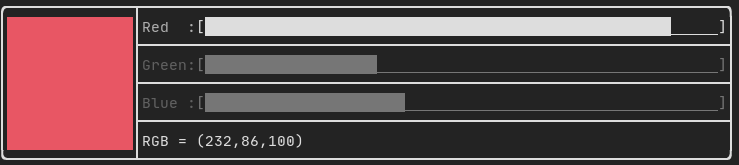
Produced by: ftxui::Slider()
from "ftxui/component/component.hpp"
Renderer
Produced by: ftxui::Renderer()
from ftxui/component/component.hpp. This component decorate another one by using a different function to render an interface.
Example:
auto inner = [...]
auto renderer = Renderer(inner, [&] {
return inner->Render() | border
});
ftxui::Renderer
also supports the component decorator pattern:
auto component = [...]
component = component
| Renderer([](
Element e) {
return e | border))
| Renderer(bold)
As a short hand, you can also compose a component with an element decorator:
auto component = [...]
component = component | border | bold;
CatchEvent
Produced by: ftxui::CatchEvent()
from ftxui/component/component.hpp. This component decorate others, catching events before the underlying component.
Examples:
auto screen = ScreenInteractive::TerminalOutput();
auto renderer = Renderer([] {
return text("My interface");
});
auto component = CatchEvent(renderer, [&](
Event event) {
if (event == Event::Character('q')) {
screen.ExitLoopClosure()();
return true;
}
return false;
});
screen.Loop(component);
The ftxui::CatchEvent
can also be used as a decorator:
component = component
| CatchEvent(handler_1)
| CatchEvent(handler_2)
| CatchEvent(handler_3)
;
Collapsible
Useful for visual elements whose visibility can be toggle on/off by the user. Essentially, this the combination of the ftxui::Checkbox()
and ftxui::Maybe()
components.
auto collabsible = Collapsible("Show more", inner_element);
Maybe
Produced by: ftxui::Maybe()
from ftxui/component/component.hpp. This component can be utilized to show/hide any other component via a boolean or a predicate.
Example with a boolean:
bool show = true;
auto component = Renderer([]{ return "Hello World!"; });
auto maybe_component = Maybe(component, &show)
Example with a predicate:
auto component = Renderer([]{ return "Hello World!"; });
auto maybe_component = Maybe(component, [&] { return time > 10; })
As usual, ftxui::Maybe
can also be used as a decorator:
component = component
| Maybe(&a_boolean)
| Maybe([&] { return time > 10; })
;
Container
Horizontal
Produced by: ftxui::Container::Horizontal()
from "ftxui/component/component.hpp". It displays a list of components horizontally and handle keyboard/mouse navigation.
Vertical
Produced by: ftxui::Container::Vertical()
from "ftxui/component/component.hpp". It displays a list of components vertically and handles keyboard/mouse navigation.
Tab
Produced by: ftxui::Container::Tab()
from "ftxui/component/component.hpp". It take a list of component and display only one of them. This is useful for implementing a tab bar.
Vertical:
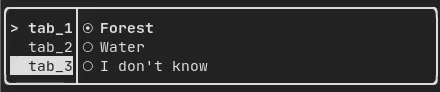
Horizontal:
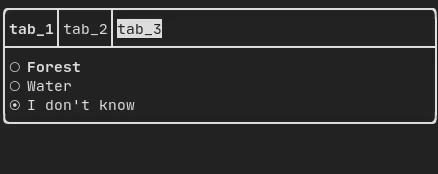
ResizableSplit
It defines a horizontal or vertical separation between two children components. The position of the split is variable and controllable using the mouse. There are four possible splits:
Example:
Force a frame redraw.
Typically, ftxui::ScreenInteractive::Loop()
is responsible for drawing a new frame whenever a new group of events (e.g keyboard, mouse, window resize, etc.) has been processed. However, you might want to react to arbitrary events that are unknown to FTXUI. To accomplish this, you must post events using ftxui::ScreenInteractive::PostEvent
(this is thread safe) via a thread. You will have to post the event ftxui::Event::Custom
.
Example:
screen->PostEvent(Event::Custom);